January 31, 2024 • For devs
Unraveling Debugging Methods in JavaScript: Techniques Every Developer Should Know
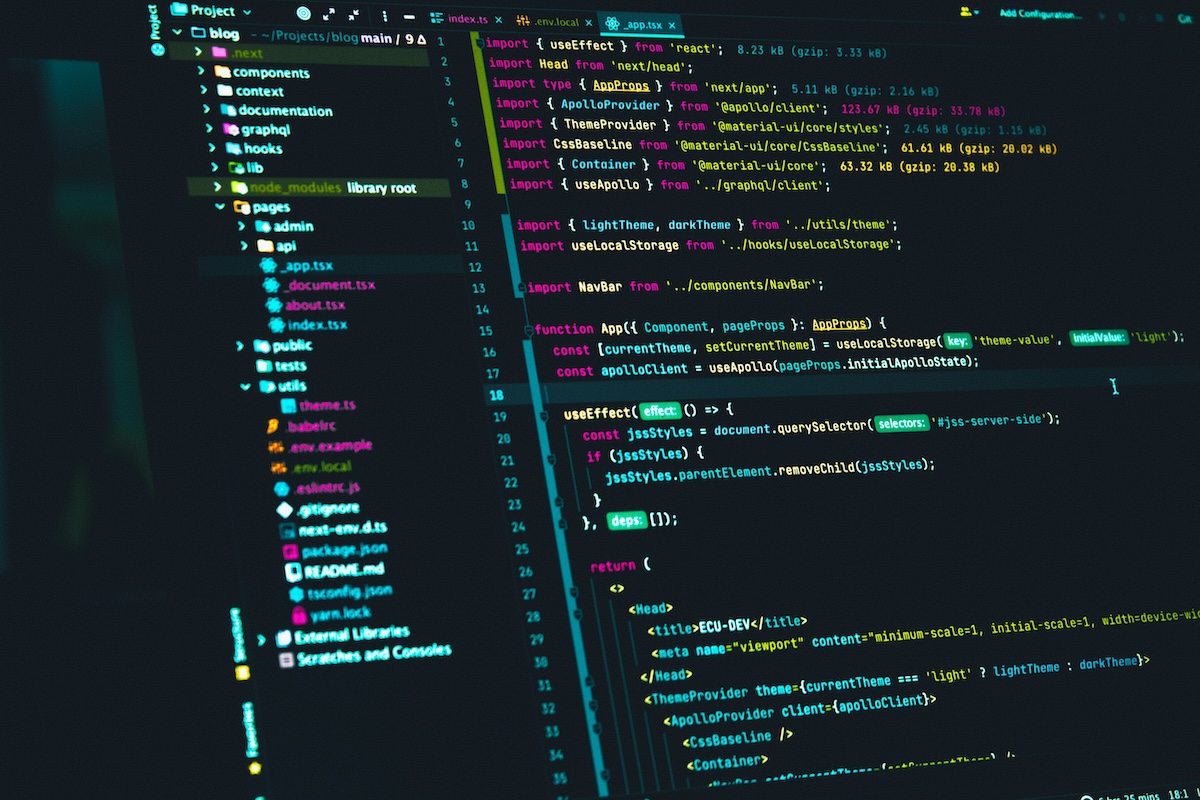
We're Streply, a tool that tracks errors and manages logs quickly. It's easy to set up and compatible with many popular frameworks. Made by developers, for developers! If you need to keep an eye on errors and follow logs in your app, create free account.
In the ever-evolving landscape of web development, JavaScript stands as a cornerstone, powering the dynamic behavior in the majority of modern websites. However, writing JavaScript code is not without its challenges; errors are not just probable, they are inevitable. This is where the art of debugging comes into play—an essential skill for any programmer looking to polish their code and enhance user experiences. In this comprehensive guide, we'll explore the various debugging methods in JavaScript, and offer best practices and tools to streamline this critical process.
Understanding the Importance of JavaScript Debugging
The road to flawless code is paved with bugs. Whether you're a beginner or an experienced developer, encountering errors is a natural part of the coding journey. Debugging is much more than a clean-up exercise; it's a diagnostic process that requires critical thinking, a methodical approach, and a deep understanding of the debugging tools at your disposal.
The Inevitability of Errors in Code
Writing new computer code often comes with its fair share of syntax and logical errors. Diagnosis can be tricky, especially when there are no error messages pointing you in the right direction. In JavaScript, this silent failure can lead to hours of frustration and confusion. Recognizing the types of errors and knowing how to track them is the first step in mastering JavaScript debugging.
What is Debugging?
Simply put, debugging is the process of finding and fixing issues within your code. It encompasses a holistic approach that includes testing, locating sources of errors, and correcting them to ensure that your program functions as intended. It's a systematic activity that can turn chaotic if not handled with the right tools and mindset.
JavaScript Debuggers: Your First Line of Defense
Fortunately, modern browsers are equipped with their own built-in JavaScript debuggers. These powerful tools can be toggled on and off, allowing you to view errors as they occur. With a debugger, setting breakpoints becomes effortless, giving you the opportunity to pause code execution and inspect variables in real-time.
Activating Browser Debugging Tools
The common way to access the debugging tools in most browsers is as follows:
- Chrome: Open the browser, select "More tools" from the menu, choose "Developer tools," and then select "Console."
- Firefox: Open the browser, choose "Web Developer" from the menu, and select "Web Console."
- Edge: Open the browser, select "Developer Tools" from the menu, and choose "Console."
- Opera: Open the browser, navigate to "Developer," select "Developer tools," and finally "Console."
- Safari: Go to "Safari, Preferences, Advanced" in the main menu, check "Enable Show Develop menu in menu bar," then select "Show Error Console" from the new "Develop" menu option.
The console.log() Method and Its Power
One of the simplest yet most effective debugging methods is using console.log(). This output function allows you to display JavaScript values in the browser's developer console. By strategically placing console.log() statements throughout your code, you can monitor variable values and the flow of execution, which can provide invaluable insights when hunting down elusive bugs.
// Example of using console.log()
var a = 5;
var b = 6;
var c = a + b;
console.log(c); // Outputs 11 to the console
Setting Breakpoints to Inspect Code Execution
Breakpoints are a fundamental component of any debugging strategy. They allow you to halt the execution of your JavaScript code at specific points, enabling careful inspection of your program's state at that moment. Debugging tools provide an intuitive interface to manage breakpoints, letting you step through code line by line if necessary, which is crucial for complex debugging scenarios.
Employing the debugger Keyword
JavaScript offers a debugger keyword, which acts as a programmatically set breakpoint. When the debugger encounters this keyword and the debugger is turned on, it'll pause execution, much like a manual breakpoint.
// Using the debugger keyword
var x = 5;
var y = 6;
debugger;
var z = x + y;
In the above snippet, the execution will pause before calculating z, allowing you to examine the values of x and y.
Best Practices in JavaScript Debugging
When it comes to effective debugging, having a methodical approach is key. Here are some best practices to implement:
- Understand the Code: Before setting breakpoints or adding console logs, make sure you thoroughly understand what the code is meant to do. This will help you to create a mental model of expected behavior.
- Break Down the Problem: If you're facing a complex issue, break it down into smaller, more manageable chunks. Debug them independently to isolate the problem.
- Check for Common Errors: Look for typos, missing semicolons, unclosed braces or parentheses, and incorrect use of variables.
- Use Version Control: Implement a version control system such as Git. This allows you to keep track of changes and revert to previous states if a new bug is introduced.
- Keep the Console Clean: Start with a clear console. Address errors and warnings as they appear to avoid a cascade of related issues later on.
- Refine with Tools: In addition to the browser's debuggers, there are advanced tools and extensions available that can provide greater insight into your code's execution.
The Best Tools for Debugging JavaScript
While the built-in debuggers in browsers are incredibly useful, there are external tools that can enhance your debugging experience:
- Integrated Development Environments (IDEs): Programs like WebStorm or Visual Studio Code offer powerful debugging features integrated directly into the coding environment.
- Linting Tools: Tools like ESLint can help catch errors before they become bugs by statically analyzing your code for patterns that are likely to lead to errors.
- Performance Profiling: For bugs related to performance, using the profiler in your browser’s developer tools can help identify bottlenecks in code execution.
Debugging a JavaScript Codebase: Common Methods
Debugging a pre-existing JavaScript codebase often requires a more evolved strategy. Here are some methods commonly used by developers:
- Code Reviews: Collaborate with peers in reviewing code to catch bugs that you might have missed.
- Automated Testing: Implementing unit tests, integration tests, and end-to-end tests can help catch bugs early in the development cycle.
- Logging: While console.log() is handy during development, in a production environment, a robust logging system can capture errors as they occur, which can be invaluable for post-release debugging.
Conclusion: Mastering the Craft of Debugging
JavaScript debugging is a nuanced and essential skill, integral to the development of high-quality web applications. It requires patience, precision, and a well-structured approach. By utilizing built-in browser tools, embracing best practices, and leveraging additional resources, programmers can significantly reduce the time and effort spent on debugging.
Finally, it's vital to continuously stay informed about the latest debugging techniques and tools. Technology is always advancing, and so too should our approaches to tackling the challenges it presents. Embrace debugging as a core competency, and you’ll find that with each resolved issue, your skills will sharpen, leading to more robust and reliable codebases.
Remember, the first computer bug was a literal bug trapped in the electronics, but today's bugs require a modern debugger to tease out. Happy debugging!
As we delve further into the topic of JS debugging, it's important to highlight the role of consistency in your debugging strategy. Creating a stable and consistent workflow not only streamlines the debugging process but also minimizes the time you spend identifying and resolving issues.
- We are not pushy
- We only send a few emails every month. That's all.
- No spam
- We only send articles, and helpful tips for developers, not SPAM.